在Vue.js应用中,组件间的参数传递是一个常见的需求。Vue提供了多种方法来实现这一点,包括使用props、事件、Vuex、以及Vue 3中的Composition API等。以下是几种常用的方法:
1. 使用 Props
父组件可以通过props
向子组件传递数据。
父组件 (ParentComponent.vue):
<template>
<div>
<ChildComponent :message="parentMessage" />
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
parentMessage: 'Hello from Parent!'
};
}
};
</script>
子组件 (ChildComponent.vue):
<template>
<div>{{ message }}</div>
</template>
<script>
export default {
props: {
message: {
type: String,
required: true
}
}
};
</script>
2. 使用自定义事件
子组件可以通过自定义事件向父组件发送数据。
子组件 (ChildComponent.vue):
<template>
<button @click="sendMessage">Send Message to Parent</button>
</template>
<script>
export default {
methods: {
sendMessage() {
this.$emit('message-to-parent', 'Hello from Child!');
}
}
};
</script>
父组件 (ParentComponent.vue):
<template>
<div>
<ChildComponent @message-to-parent="handleMessageFromChild" />
<p>Message from Child: {{ childMessage }}</p>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
data() {
return {
childMessage: ''
};
},
methods: {
handleMessageFromChild(message) {
this.childMessage = message;
}
}
};
</script>
3. 使用 Vuex
对于大型应用,使用Vuex进行状态管理是一个好选择。Vuex允许你在组件之间共享状态。
安装Vuex:
npm install vuex
创建Vuex Store (store.js):
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex);
export default new Vuex.Store({
state: {
sharedMessage: 'Hello from Vuex!'
},
mutations: {
updateMessage(state, newMessage) {
state.sharedMessage = newMessage;
}
},
actions: {
changeMessage({ commit }, newMessage) {
commit('updateMessage', newMessage);
}
},
getters: {
getMessage: state => state.sharedMessage
}
});
在Vue实例中使用Store (main.js):
import Vue from 'vue';
import App from './App.vue';
import store from './store';
new Vue({
store,
render: h => h(App)
}).$mount('#app');
组件中使用Vuex:
<!-- ParentComponent.vue -->
<template>
<div>
<p>Message from Vuex: {{ sharedMessage }}</p>
<button @click="changeMessage">Change Message</button>
</div>
</template>
<script>
export default {
computed: {
sharedMessage() {
return this.$store.getters.getMessage;
}
},
methods: {
changeMessage() {
this.$store.dispatch('changeMessage', 'New Message from Parent!');
}
}
};
</script>
<!-- ChildComponent.vue -->
<template>
<div>
<p>Message from Vuex: {{ sharedMessage }}</p>
<button @click="changeMessage">Change Message from Child</button>
</div>
</template>
<script>
export default {
computed: {
sharedMessage() {
return this.$store.getters.getMessage;
}
},
methods: {
changeMessage() {
this.$store.dispatch('changeMessage', 'New Message from Child!');
}
}
};
</script>
4. 使用 Vue 3 的 Composition API
Vue 3引入了Composition API,提供了一种新的方法来组织和重用逻辑。
父组件 (ParentComponent.vue):
<template>
<div>
<ChildComponent :sharedState="sharedState" @updateState="updateState" />
<p>Message from Child: {{ sharedState.message }}</p>
</div>
</template>
<script>
import { ref } from 'vue';
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
setup() {
const sharedState = ref({ message: 'Hello from Parent!' });
function updateState(newState) {
sharedState.value = newState;
}
return {
sharedState,
updateState
};
}
};
</script>
子组件 (ChildComponent.vue):
<template>
<div>
<input v-model="localMessage" />
<button @click="sendMessageToParent">Send Message to Parent</button>
</div>
</template>
<script>
import { ref, watch } from 'vue';
export default {
props: {
sharedState: {
type: Object,
required: true
}
},
setup(props, { emit }) {
const localMessage = ref(props.sharedState.message);
watch(localMessage, (newValue) => {
emit('updateState', { message: newValue });
});
function sendMessageToParent() {
emit('updateState', { message: localMessage.value });
}
return {
localMessage,
sendMessageToParent
};
}
};
</script>
这些方法各有优缺点,选择哪一种方法取决于你的具体需求和项目的复杂度。对于简单的父子通信,props
和事件通常是足够的。对于更复杂的状态管理,Vuex或Composition API可能是更好的选择。
学在每日,进无止境!更多精彩内容请关注微信公众号。
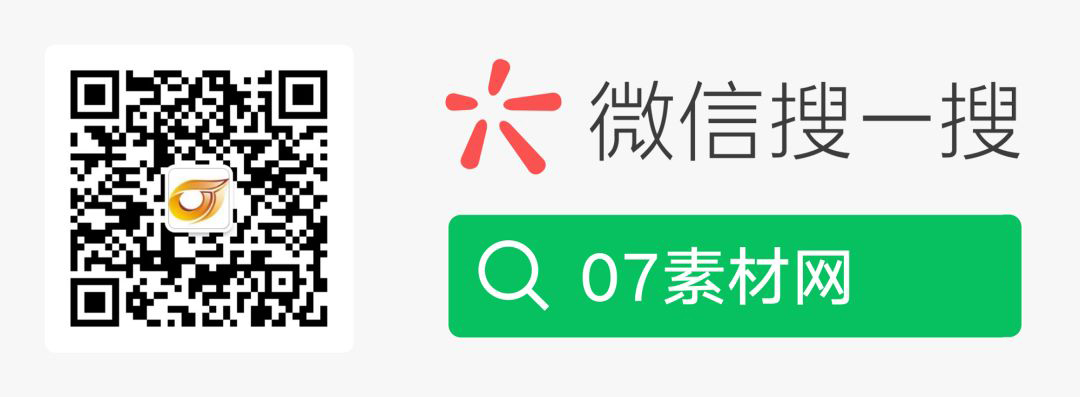
原文出处:
内容由AI生成仅供参考,请勿使用于商业用途。如若转载请注明原文及出处。
出处地址:http://www.07sucai.com/tech/229.html
版权声明:本文来源地址若非本站均为转载,若侵害到您的权利,请及时联系我们,我们会在第一时间进行处理。